A faster and simpler bootstrap menu
Based on pure CSS and Vanilla JavaScript

A lightning-fast bootstrap menu with no dependencies
Most webmasters that come to me for Core Web Vitals support use jQuery in combination with a framework such as Bootstrap.
To take full advantage of bootstrap, you will have to add no less than 3 scripts. jQuery, popper and bootstrap.
<script src="/js/jquery.js"></script>
<script src="/js/popper.js"></script>
<script src="/js/bootstrap.js"></script>
These scripts take time to download and evaluate. This is practically always at the expense of the 'Time To Interactive' and, depending on how the page is built, also at the expense of other lighthouse metrics such as First Contentful Paint and Largest Contentful Paint.
I'm going to show you how to fix this by using only CSS and Vanilla JavaScript with no dependencies. For your convenience, I have simplified the examples to make them more easy to follow.
Mobile navigation
Bootstrap uses its own JavaScript for mobile navigation. To me that always seemed like overkill. You can have a mobile navigation hamburger menu without JavaScript. The code is actually very simple. I will use an invisible checkbox element and the CSS subsequent-sibling combinator to open and close the menu element via CSS.
As soon as you I on the hamburger menu, the checkbox becomes active and that leads to the menu being displayed.
The HTML code
<nav>
<input type="checkbox" id="real-navbar-toggle">
<label for="real-navbar-toggle">☰</label>
<ul>
<li>
<a href="#">Home</a>
</li>
</ul>
</nav>
CSS code
#real-navbar-toggle{display:none;}
#real-navbar-toggle:checked ~ ul{display:block;}
The result
Dropdown menu's
Dropdown menus are a bit trickier. Of course I can also use the hidden checkbox element. But that often leads to difficulties in your CMS where menus are created automatically. That's why I will use JavaScript for this. Only the code that I am using is much faster and doesn't require any dependencies such as jQuery. You can use this script as a drop-in for your current scripts if you like.
The HTML code
<nav>
<div>Logo</div>
<ul class="navbar-nav">
<li>
<a href="#">Home</a>
</li>
<li>
<a class="dropdown-toggle" href="#">
Dropdown toggle
</a>
<div>
<a href="#">Link 1</a>
<a href="#">Link 2</a>
</div>
</li>
</ul>
</nav>
The JavaScript code
var elements = document.getElementsByClassName("dropdown-toggle");
for (var i = 0; i < elements.length; i++) {
elements[i].addEventListener("click", function(e) {
e.preventDefault();
for (var y = 0; y < elements.length; y++) {
if (elements[y] == this) {
elements[y].nextElementSibling.classList.toggle("show");
} else {
elements[y].nextElementSibling.classList.remove("show");
}
}
});
}
The result
Need your site lightning fast?
Join 500+ sites that now load faster and excel in Core Web Vitals.
- Fast on 1 or 2 sprints.
- 17+ years experience & over 500 fast sites
- Get fast and stay fast!
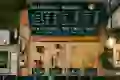
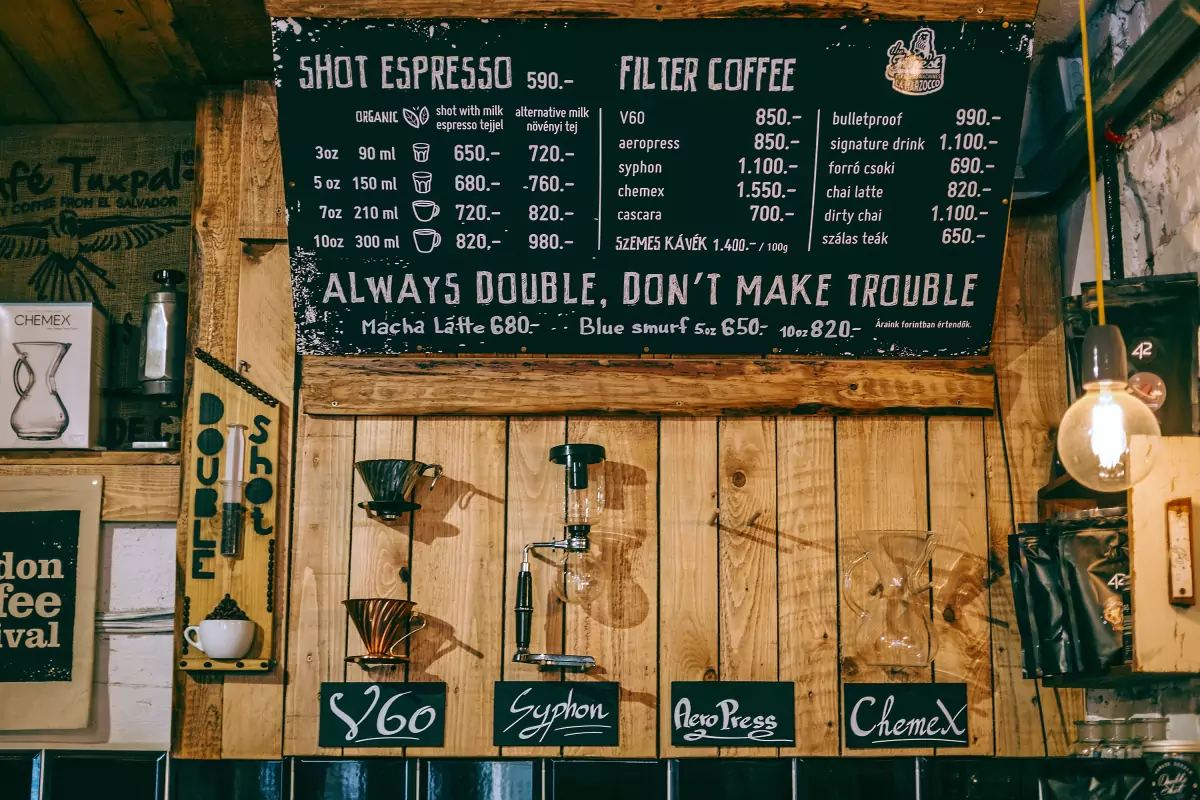